What Is jQuery append()?

jQuery is an easy-to-use open-source JavaScript library. jQuery provides various methods to add interactive functionalities into your website which makes it unique from other JavaScript libraries. The jQuery append() method is used to append text or content specified by the parameter to the end of each element in the set of matched elements.
What Does jQuery append() Do?
The jQuery append() method appends or inserts the specified content as the last child by the parameter, to the end of each element in the set of matched elements. The .append() and .appendTo() methods performs the same task. The only difference is that with the append() method the selector expression preceding the method is the container into which the content is appended while in the appendTo() method the content precedes the method, either as a selector expression or as markup created, and it is appended into the target container.
How Does jQuery append() Work?
The jQuery append() is used to insert the content at the end of the selected elements.
The syntax of append() is as follows:
$(selector).append(content,function(index,html))
OR $(selector).append( content [, content ] [, content ] [, content ]..... )
The “content” specifies the content to append or insert. The “function” specifies a function that returns the content to insert where “index” returns the index position of the element in the set and “Html” returns the current HTML of the selected element.
You can append any number of content within a single append statement. You can create several new elements with HTML, Text, Array, jQuery, and DOM. And then you can append these elements to the text using the append() method.
Let's take a simple example to append text on the list.
html>
<head>
<script
src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("#btn2").click(function(){
$("ol").append("<li>Text New(Appended Text)</li>");
});
});
</script>
</head>
<body>
<h2>
<p>Example to Append Text using .append() Method</p>
</h2>
<ol>
<li>Text 1</li>
<li>Text 2</li>
<li>Text 3</li>
</ol>
<button id="butn2">Append Text</button>
</body>
</html>
This is what the output for the above code will be. You can explore it some more on your own using CodePen.

What Are The Ways To Append Elements Using The append() Method?
There are two ways to insert an element using the append() method:
- Append content using the append() method
- Append content using a function
1.Append Content using the append() method:
In this method, you can create content using HTML, Text, Array, jQuery, and DOM and append it by defining elements directly inside the append() method.
Let's take a short example to understand how this method works:
<!DOCTYPE html>
<html>
<head>
<script
src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
function appendText() {
var txt1 = "<p>Text1</p>"; // Create text with HTML
var txt2 = $("<p></p>").text("Text2"); // Create text with jQuery
var txt3 = document.createElement("p");
txt3.innerHTML = "Text3"; // Create text with DOM
$("body").append(txt1, txt2, txt3); // Append new elements
}
</script>
</head>
<body>
<h2>
<p>Append Content Using .append() Method </p>
</h2>
<button onclick="appendText()">Append Text</button>
</body>
</html>
Here's how the output will look for the above code:
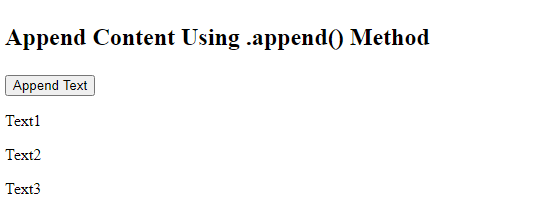
2.Append content using a function
This method is used to append a text or content using a function defined inside the <script> tag. It returns the content to append at the end of each element in the set of matched elements.
Let's take an example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("p").append(function(n){
return "<b>Appened Text " + n + ".</b>";
});
});
});
</script>
</head>
<body>
<h1>Append Content Using Function</h1>
<p>This is </p>
<p>This is </p>
<button>Insert Content at the end of each "p" element</button>
</body>
</html>
This is what the output will be:

Conclusion
In this tutorial, we learned how to use jQuery append(). We saw how it can be very helpful to append content or text. We also saw some of the different ways to use jQuery append(). It is interesting to know that jQuery also has a function called appendTo() in its library. Even though these are similar functions, they have a difference between, especially in their syntax.
To keep learning such interesting facts about jQuery, subscribe to our blog!
To learn more about jQuery find(), visit our previous blog!