What Are jQuery Effects?

jQuery is the most used JavaScript library for designing a variety of web pages. Designing web pages not only includes content and styles but also various effects and animations to make it attractive.
In order to design effective web pages for your website, jQuery provides various effects and animations. It easily adds various effects and animations to your web pages just within a few steps.
In this tutorial, we will learn what jQuery effects do and the different types of effects that can be added using jQuery functions. Some of the effects we will learn today are Hide/Show effect, fadeIn and fadeOut effects and some others.
Let's dive in to the topic!
What Does jQuery Effects Do?
jQuery Effects are like the cherry on the cake in designing web pages. It adds effectiveness and helps to easily create web pages with various effects and animations.
You can add various effects like hide/show, fade, slide, animate, toggle, and much more. jQuery allows you to add simple, standard effects that are commonly used, and the ability to create new custom effects.
How To Use The jQuery Effects?
You can quickly
add jQuery effects and animations to your HTML code. The effects are added by including
jQuery effect methods into your <script> tag.
Let us see an
example to use the jQuery Hide/Show Effect. Here when you will click on the
“Hide” button the text will disappear and when you click on the “Show” button
the text will appear again.
Code:
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#hide").click(function(){
$("p").hide();
});
$("#show").click(function(){
$("p").show();
});
});
</script>
</head>
<body>
<h1>Example To Use jQuery Hide/Show Effect</h1>
<p>Try Me!</p>
<button id="hide" style="background-color: lightblue">Hide</button>
<button id="show" style="background-color: lightblue">Show</button>
</body>
</html>
You can see the hide and show effect in the output below:
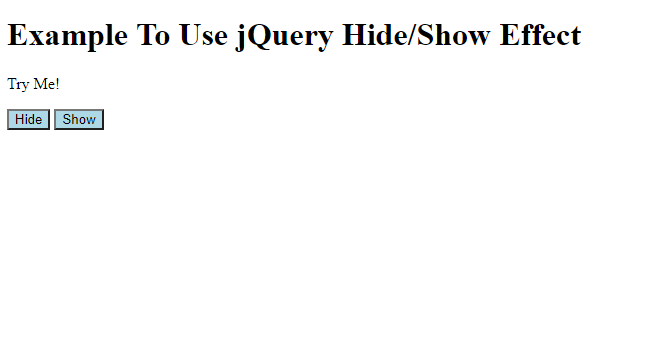
What Are The Various jQuery Effect Methods?
jQuery provides a lot of standard effect methods to design beautiful web pages. Learning these effects can can be really helpful for a web developer to create interactive web pages. Some of the methods are as follows:
1.animate()
It is used to
run custom animations on the selected elements.
Syntax: (selector).animate({styles},speed,easing,callback)
Here “styles”
specifies one or more CSS values to animate, “speed” specifies the speed of to animate,
“easing” also determines speed but at different points in animation, and
“callback” is to be executed after the animation completes.
2.delay()
This method is
used to delay the execution of all queued functions on the selected elements.
Syntax: $(selector).delay(speed,queueName)
Here
“queueName” specifies the name of the queue.
3.fadeIn()
It is used to
fade in the selected elements.
Syntax: $(selector).fadeIn()
4.fadeOut()
It is used to
fade out the selected elements.
Syntax: $(selector).fadeOut()
5.hide()
This method is
used to hide the selected elements.
Syntax: $(selector).hide()
6.show()
This method is
used to show the selected elements.
Syntax: $(selector).show()
7.slideUp()
It is used to
slide-up or hide the selected elements.
Syntax: $(selector).slideUp()
8.stop()
This method
stops the currently running effect or animation for the selected elements.
Syntax: $(selector).stop()
9.toggle()
It toggles
between the hide() and show() methods.
Syntax: $(selector).toggle()
Let us see an
example using the fadeIn Effect. Here when you will click on the button the
content will fade.
Code:
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#box1").fadeIn();
$("#box2").fadeIn("slow");
$("#box3").fadeIn(3000);
});
});
</script>
</head>
<body>
<h1>Example To Use jQuery fadeIn Effect</h1>
<button>Click Me to fadeIn Content</button><br><br>
<div id="box1" style="width:90px;height:50px;display:none;background-color: lightblue;"><h2>jQuery</h2></div><br>
<div id="box2" style="width:90px;height:50px;display:none;background-color:lightblue;"><h2>fadeIn</h2></div><br>
<div id="box3" style="width:90px;height:50px;display:none;background-color: lightblue;"><h2>Effect</h2></div>
</body>
</html>
Here's how the output will be:
Before Click

After Click

Conclusion
In this tutorial, we learned what is jQuery effects and its various types. We also learned how to use various jQuery effects. In the above examples, we implemented the jQuery Hide/Show effect to hide/show contents as well as jQuery fadeIn effect to fadeIn the contents.
You can also use other jQuery effects like this for designing your web pages. To keep learning such other effects, subscribe to our blog! To learn what is jQuery find, visit our previous article!